##Loading the image
Load an image by calling the static load
method on the Image
and passing in the $pathToImage
.
use Spatie\Image\Image;
$image = Image::load(string $pathToImage);
##Selecting a driver
By default, the Imagick driver will be used. However if you would like to use GD you can do this by selecting the driver before loading the image.
use Spatie\Image\Image;
Image::useImageDriver(ImageDriver::Gd)->loadFile(string $pathToImage);
It's also possible to pass an implementation of ImageDriver
directly. Build your own driver from scatch, or extend one of the provided drivers (ImagickDriver
or GdDriver
).
use Spatie\Image\Image;
Image::useImageDriver(MyDriver::class)->loadFile(string $pathToImage);
##Applying manipulations
Any of the image manipulations can be applied to the loaded Image
by calling the manipulation's method. All image manipulation methods can be chained.
use Spatie\Image\Image;
Image::load('example.jpg')
->sepia()
->blur(50)
->save();
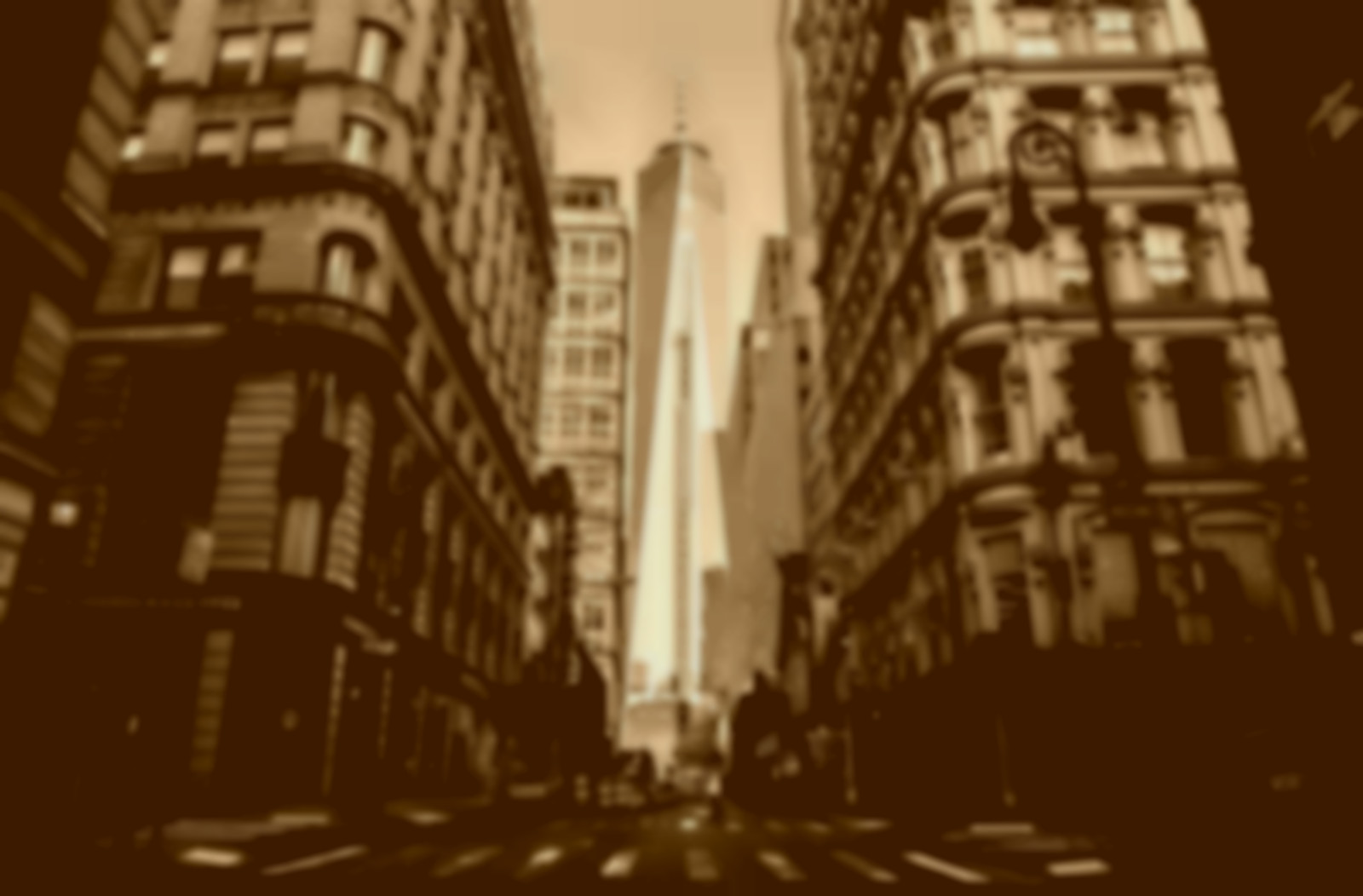
Every manipulation you call will be applied. When calling a manipulation method multiple times each call will be applied immediately.
use Spatie\Image\Image;
Image::load('example.jpg')
->brightness(-40)
->brightness(-20)
->save();
##Saving the image
Calling the save
method on an Image
will save the modifications to the specified file.
use Spatie\Image\Image;
Image::load('example.jpg')
->width(50)
->save('modified-example.jpg');
To save the image in a different image format or with a different jpeg quality see saving images.
##Retrieve a base64 string
Calling the base64
method on an Image
will return a base64 string of the image.
use Spatie\Image\Image;
Image::load('example.jpg')
->base64();
By default the base64 string will be formatted as a jpeg and will include the mime type.
You can alter this by passing extra parameters:
Image::load('example.jpg')
->base64('jpeg', $prefixWithFormat = false);