##Width and height
The width and height of the Image
can be modified by calling the width
and height
functions and passing in the desired dimensions in pixels. The resized image will be contained within the given $width
and $height
dimensions respecting the original aspect ratio.
$image->width(int $width);
$image->height(int $height);
##Example usage
Image::load('example.jpg')
->width(250)
->height(250)
->save();
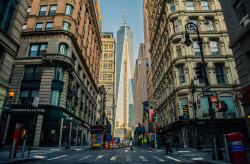
The fit
method fits the image within the given $width
and $height
dimensions (pixels) using a certain $cropMethod
.
$image->fit(string $cropMethod, int $width, int $height);
The following $cropMethod
s are available through constants of the Manipulations
class:
##Manipulations::FIT_CONTAIN
This is the default fitting method. The image will be resized to be contained within the given dimensions respecting the original aspect ratio.
##Manipulations::FIT_MAX
The image will be resized to be contained within the given dimensions respecting the original aspect ratio and without increasing the size above the original image size.
##Manipulations::FIT_FILL
Like FIT_CONTAIN
the image will be resized to be contained within the given dimensions respecting the original aspect ratio. The remaining canvas will be filled with a background color.
$image
->fit(Manipulations::FIT_FILL, 497, 290)
->background('007698');
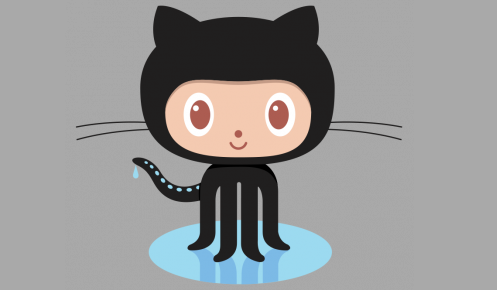
##Manipulations::FIT_STRETCH
The image will be stretched out to the exact dimensions given.
##Manipulations::FIT_CROP
The image will be resized to completely cover the given dimensions respecting the orginal aspect ratio. Some parts of the image may be cropped out.
##Example usage
Image::load('example.jpg')
->fit(Manipulations::FIT_STRETCH, 450, 150)
->save();
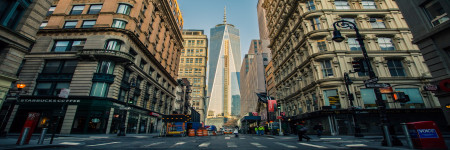
By calling the crop
method part of the image will be cropped to the given $width
and $height
dimensions (pixels). Use the $cropMethod
to specify which part will be cropped out.
$image->crop(string $cropMethod, int $width, int $height);
The following $cropMethod
s are available through constants of the Manipulations
class:
CROP_TOP_LEFT
, CROP_TOP
, CROP_TOP_RIGHT
, CROP_LEFT
, CROP_CENTER
, CROP_RIGHT
, CROP_BOTTOM_LEFT
, CROP_BOTTOM
, CROP_BOTTOM_RIGHT
.
##Example usage
Image::load('example.jpg')
->crop(Manipulations::CROP_TOP_RIGHT, 250, 250)
->save();
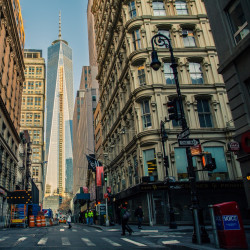
##Focal crop
The focalCrop
method can be used to crop around an exact position. The center of the crop is controlled by the $focalX
and $focalY
values in percent (0
- 100
).
You can also zoom into your focal point, if needed. Zoom is controlled by a floating point ranging from 1
to 100
. Each step represents a 100% zoom, so passing 2 will be the same as viewing the image at 200%. The suggested range is 1-10.
$image->focalCrop(int $width, int $height, int $focalX, int $focalY, float $zoom = 1);
##Manual crop
The manualCrop
method crops a specific area of the image by specifying the $startX
and $startY
positions and the crop's $width
and $height
in pixels.
$image->manualCrop(int $width, int $height, int $x, int $y);
##Example usage
Image::load('example.jpg')
->manualCrop(600, 400, 20, 620)
->save();
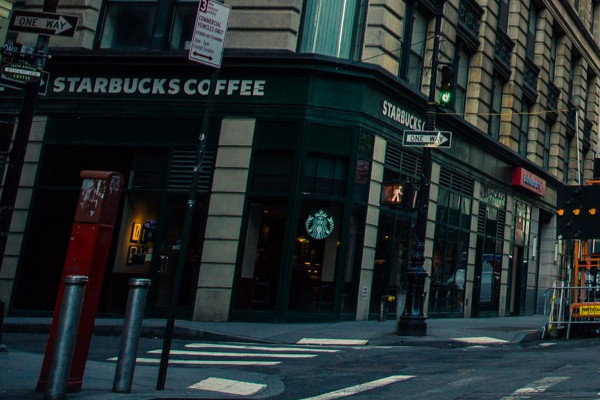